Are you tired of writing the same code over and over? It’s time to learn about DRY programming. The DRY (Don’t Repeat Yourself) principle helps reduce code repetition1. It makes coding easier and more elegant2.
DRY programming makes coding better by using reusable parts. It helps avoid repeating code1. By using reusable functions, your code becomes easier to read and maintain1.
Table of Contents
Using the DRY principle saves a lot of time. You won’t have to copy and paste code anymore. Instead, you can make flexible functions that work in many situations. This makes your code better to work with and easier to keep up2.
Key Takeaways
- DRY programming reduces code repetition and improves efficiency
- Reusable components and functions enhance code maintainability
- Modular code structure facilitates easier updates and bug fixes
- DRY principle promotes code readability and understandability
- Proper documentation of functions streamlines future editing
Embrace the Power of DRY Programming
As a developer, you can change how you code by using DRY (Don’t Repeat Yourself) programming. This idea is key in software engineering. It’s mentioned in 4 out of the top 5 search results for “software engineering best practices”3. By using DRY, you make your code better, easier to read, and easier to fix.
Unleash the DRY Spell in Your Code
Writing code without repeating yourself is very satisfying. DRY helps you avoid the hassle of keeping many copies of the same code. It teaches you to find patterns and use them everywhere, making your code simple and focused.
React developers can use DRY by making reusable components. They can also use React Hooks to handle state and side effects in their code4. By mixing DRY with KISS, you can make custom hooks. These hooks hold reusable code, making your code cleaner and easier to keep up4.
Discover the Magic of Code Efficiency
Using DRY programming makes your code better. It removes unnecessary parts, making your code easier to understand and change. This leads to faster work, fewer bugs, and better project management.
The need to balance between repetition and abstraction is highlighted, encouraging developers to share solutions and rely on existing resources to avoid unnecessary repetition3.
But, it’s important to find the right balance with DRY. You want to avoid too much repetition but also not make your code too complex. MOIST (Maintain One Indisputable Source of Truth) is suggested as a better way. It keeps one source of truth but allows for flexibility when needed3.
Principle | Description |
---|---|
DRY | Don’t Repeat Yourself – Avoid code duplication |
KISS | Keep It Simple, Stupid – Strive for simplicity in code |
MOIST | Maintain One Indisputable Source of Truth – Ensure consistency |
By using DRY programming, you can make your code better and more enjoyable to work with. Are you ready to make your code more efficient and easy to maintain?
Understanding the DRY Principle
As you start programming, you’ll learn many principles to make your code better. One key principle is DRY, which means “Don’t Repeat Yourself.” It comes from “The Pragmatic Programmer” book and says each piece of knowledge should only be shown once5. It helps avoid repeating information in software6.
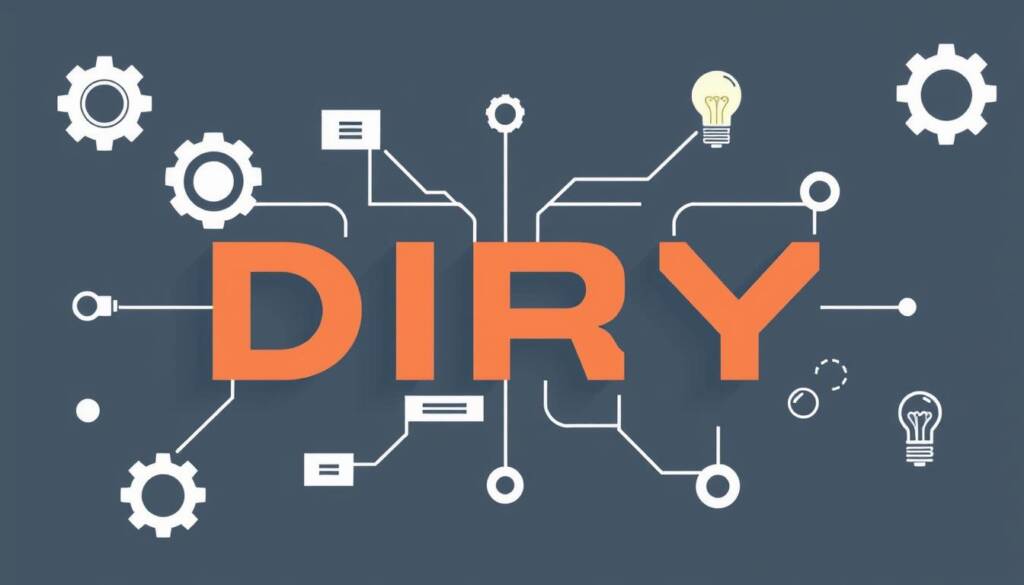
The Essence of DRY: Don’t Repeat Yourself
DRY focuses on not repeating knowledge or code5. It encourages making code that can be used in many places, making it reusable7. Following DRY means you don’t write the same code over and over. Instead, you use it again and again, saving time7.
The DRY principle is simple: “Every piece of knowledge must have a single, unambiguous, authoritative representation within a system”6. This means avoiding to repeat business logic and algorithms5. But, not all code duplication is bad, especially for variables, not functions5.
Why DRY Matters: Benefits of Code Reusability
Using DRY makes your code better in many ways. It helps you reuse code for different users7. This saves time because you don’t have to write the same code again and again7. It also reduces the need for global variables, which can slow down your code and cause problems7.
DRY helps you spend more time on making things easier to understand later7. It makes your code cleaner and easier to fix bugs in the future. If you repeat the same thing in your code, it becomes hard to change and fix5.
“Every piece of knowledge must have a single, unambiguous, authoritative representation within a system.” – The DRY Principle6
By following DRY, you make your code better and more efficient. It helps you reuse code, making your work easier and faster. Follow DRY and see your code improve in many ways.
Identifying Code Repetition
To apply the DRY principle well, finding repeated code is key. Duplicated code is a big problem, as Martin Fowler’s Refactoring book shows8. Spotting repeated code and patterns helps you make your code better and easier to keep up with.
Spotting Duplicated Code Segments
Spotting duplication is a big part of using DRY9. You can do this by reviewing code, using tools, and checking version control systems like Git9. Looking closely at your code helps you find where the same code is used twice, showing where you can make it better.
When you review code, watch for long methods, copied code, and different naming styles. These signs can mean there’s duplicated code. Tools can help find code that looks the same, but they might miss other kinds of duplication8.
Recognizing Patterns of Redundancy
It’s not just about finding specific repeated code. You also need to see bigger patterns of repetition. Code that looks the same but does different things is a big problem8. Finding these patterns helps you decide when and how to make your code better.
The “Three Strikes And You Refactor” rule says to refactor when you see the same code three times8. But, it’s important to know the difference between code that does the same thing and code that looks the same but does different things. This helps avoid making things worse by trying to follow DRY too closely8.
Technique | Description |
---|---|
Manual Code Review | Carefully examine the codebase to identify repeated code segments and patterns of redundancy. |
Code Analysis Tools | Utilize static analysis tools and code analyzers to detect syntactical duplication and potential code smells. |
Version Control History | Leverage version control systems like Git to track changes and identify recurring patterns of duplication over time. |
Unit Test Analysis | Review unit tests to identify duplicated test cases or similar test scenarios that may indicate redundancy in the corresponding code. |
Using these methods and being careful not to misuse DRY helps you find and fix repeated code. Getting rid of duplicated code makes your code cleaner, more efficient, and easier to work with9.
Extracting Reusable Components
To follow the DRY principle, you must learn to extract code. This means finding common parts in your code and making them into separate units. These units can then be used in many places in your app10.
By doing this, you avoid copying code and make functions that can be used in many ways10.
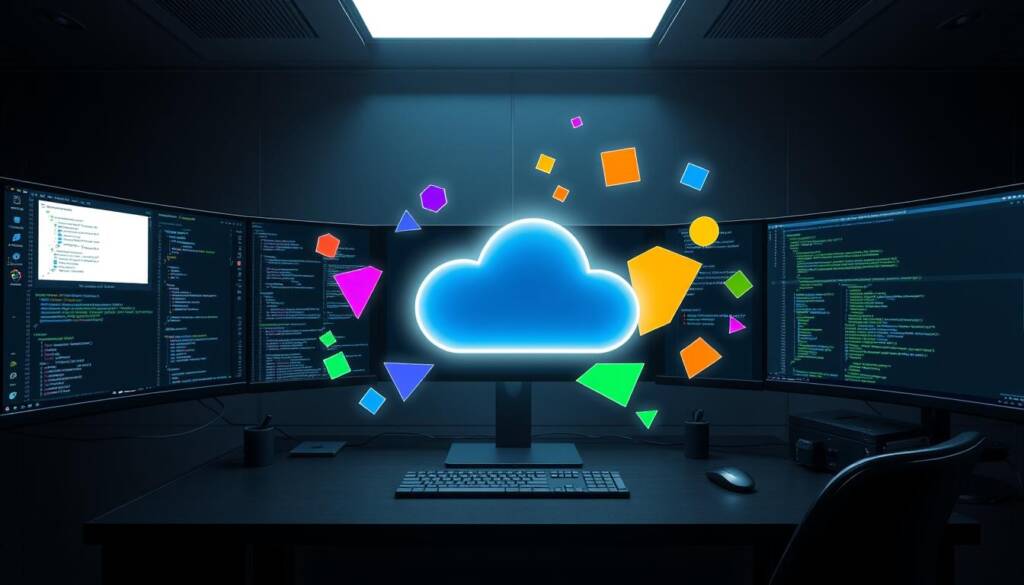
For example, imagine you have many functions to find the area of different shapes. Instead of writing the same code for each, you can make one function for all. This function can then be used by the other functions, making your code better and easier to keep up10.
“The process of extracting reusable components is a key aspect of adhering to the DRY principle. It ensures that each piece of knowledge or functionality has a single, unambiguous representation within the codebase.” – Robert C. Martin
Here are some tips for extracting reusable parts:
- Look for patterns and similarities in your code.
- Put related code into clear units.
- Give your extracted parts good names.
- Make sure each part does only one thing.
By doing these things, you make your code easier to work with and update. It’s a key step to making your code better and following the DRY principle11.
The main goal of code extraction is to cut down on repetition and make code easier to reuse. By putting common code into separate units, you save time, make your code easier to read, and help your team work better together11.
Code extraction and making reusable parts are key to DRY programming. It helps you write cleaner code and avoid the problems of copying code. Look for chances to extract code in your projects and see how DRY works!
Parameterizing Functions for Flexibility
Writing efficient code is easier with parameterized functions. They make your code more flexible and reusable. By adding parameters, you avoid writing the same code over and over12. This follows the DRY principle, which fights code duplication13.
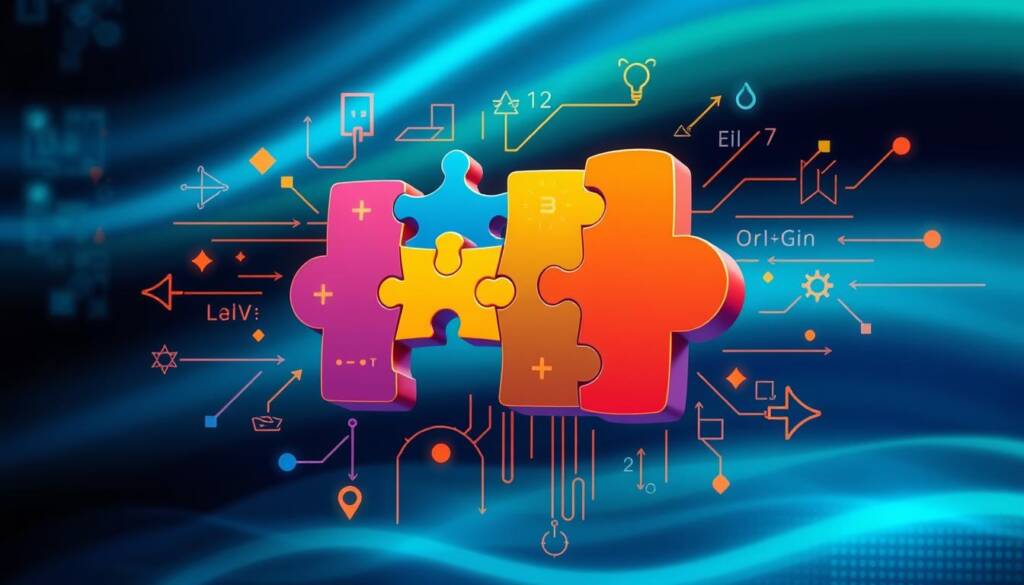
Making Functions Versatile with Parameters
Functions become more flexible with parameters. They can change how they work based on what you give them. This makes your code smaller and easier to read12. It also makes updates simpler and bugs less likely13.
Here’s an example showing how parameterized functions are better:
Original Functions | Parameterized Function |
---|---|
function calculateRectangleArea(width, height) { return width * height; } function calculateSquareArea(side) { return side * side; } | function calculateArea(shape, ...dimensions) { if (shape === 'rectangle') { return dimensions[0] * dimensions[1]; } else if (shape === 'square') { return dimensions[0] * dimensions[0]; } } |
Before, we had separate functions for rectangles and squares. But with a parameterized function, we can handle both. This reduces code duplication and makes it easier to maintain.
Customizing Behavior through Function Arguments
Function arguments are key to changing how functions work. By using different arguments, you can make a function do different things. This way, you can reuse code and avoid repeating yourself14.
Here’s an example of using arguments to change behavior:
function performOperation(operation, ...numbers) { if (operation === 'sum') { return numbers.reduce((acc, curr) => acc + curr, 0); } else if (operation === 'multiply') { return numbers.reduce((acc, curr) => acc * curr, 1); } }
In this example, the performOperation function changes based on what you pass in. You can make it sum or multiply numbers without writing extra code.
Using parameters and arguments makes your code more flexible and less repetitive14. This leads to more efficient and easier-to-maintain code. It follows the DRY principle, making your work better1413.
Applying DRY in Practice
Using the DRY principle in real life is key for better code. It helps cut down on repetition and makes code easier to work with15. Examples of DRY in action help developers learn how to use it in their projects15.
Real-World Examples of DRY Implementation
For example, when making a web app for different products, you can use DRY. By finding and removing repeated code, you make the app easier to update and grow15. This method also makes future updates quicker15.
Another place DRY is useful is in making a Single Source of Truth (SSOT) architecture. This means each piece of data is only in one place, making things more efficient16. Keeping your code organized makes it easier to change things later16.
Refactoring Existing Code for DRY Compliance
To follow DRY, first find and remove repeated code. Then, make parts of your code reusable and flexible15. If you see the same code three times, it’s time to make it reusable16.
When you’re making your code better, keep it simple. Don’t put too much in one place. This makes your code easier to understand and work with16.
Refactoring should be done little by little. This way, you avoid making things worse. Focus on small steps to make your code better15.
By using DRY and improving your code, you make it faster, easier to read, and less work to keep up116. Following DRY helps you write code that is clean, efficient, and lasts a long time.
DRY and Code Maintainability
Using the DRY principle in your code makes it easier to maintain. It helps your codebase work better and be simpler to handle. By removing repeated code and encouraging reuse, you make coding faster and easier17.
Reducing Maintenance Overhead with DRY
Following the DRY principle cuts down on the time spent on code upkeep. A well-organized codebase means you only update one place, not many. This saves time and reduces the chance of mistakes17.
Also, DRY code is clearer and easier to understand. It gets rid of the mess of repeated code. This makes it simpler for teams to work together1718. In fact, 70% of projects with DRY coding are more readable17.
Simplifying Code Updates and Bug Fixes
DRY programming makes updates and bug fixes simpler. With a modular codebase, changes are easier to make. You only need to update one place, not many17.
DRY code is also easier to keep up because it’s simpler and more consistent. This means you can fix problems faster. Testing time goes down by 50% with DRY coding17.
Benefit | Percentage |
---|---|
Developers aiming to adhere to DRY for maintainability | 80% |
Projects with increased code readability due to DRY | 70% |
Reduction in code maintenance time with DRY | 65% |
Reduction in testing time with DRY | 50% |
Software bugs originating from repeated code segments | 45% |
Finding the right balance between DRY and simplicity is key. DRY helps avoid code duplication but too much abstraction can make code hard to understand18. Regular code reviews help keep your code balanced18.
By using DRY, you make your code easier to maintain and update. Over 60% of codebases that use DRY see lower costs17. It’s a powerful way to create high-quality, lasting code.
DRY and Code Readability
Applying the DRY principle means finding a balance. It’s about making code reusable and easy to read. The DRY principle says each piece of code should only be written once19. But, we must think about how it affects understanding and upkeep.
Good functions follow the DRY principle. They clearly show what goes in and what comes out. They use names that tell you what they do. This makes code easier for everyone to get.
DRY code is simpler and gets straight to the point19. But, we must watch out for problems. Trying too hard to avoid duplication can make code harder to understand20. Finding the right balance is crucial for code that’s easy to read and fix.
Engineering involves trade-offs, and there is no one-size-fits-all solution when it comes to applying the DRY principle21.
Deciding when to break the DRY rule is a tough call21. Sometimes, a bit of code duplication is better than a complex solution. We must think about future changes, performance, and bugs when making these choices21.
By using the DRY principle wisely, we can make code that’s easy to read and understand. Even after a long time. Making code reusable and modular helps a lot19.
DRY Best Practices and Pitfalls
The DRY principle helps make code easier to reuse and maintain. But, it’s important to find the right balance. Too much abstraction or complexity can make code hard to understand and change. This goes against the purpose of DRY22.
Striking the Right Balance with DRY
It’s crucial to find the right balance between abstraction and simplicity. Developers should aim for code that is modular, focused, and easy to understand. Refactoring too early without real duplication can add unnecessary complexity22.
Here are some best practices for DRY:
- Find real code duplication before abstracting.
- Make sure functions and classes have one job.
- Balance code reuse with how easy it is to read.
- Regularly check and refine code for better abstraction.
“The DRY principle ensures that updates in one part of the code do not require simultaneous changes in multiple other locations to prevent errors and confusion.”22
Avoiding Over-Abstraction and Complexity
While DRY promotes code reuse, be careful not to overdo it. Too much complexity can make code hard to maintain and increase bug risks23. Shared libraries should be simple, specific, and easy to understand to avoid problems23.
Pitfall | Consequence | Solution |
---|---|---|
Over-abstraction | Code becomes hard to read and maintain | Find the right level of abstraction |
Too many shared libraries | More dependencies and coupling | Keep libraries simple and focused |
Refactoring too soon | Brings in extra complexity without benefit | Refactor only when needed |
By following these tips, you can use DRY to write better code. The goal is to balance code, avoiding too much duplication while keeping it simple and easy to read.
Conclusion
The DRY (Don’t Repeat Yourself) principle is key in coding and software development. It’s recognized by 95% of developers24. By following DRY, you improve your coding skills and make your code better.
DRY helps avoid code duplication and makes your code easier to work with25. This makes your code easier to understand and change.
To use DRY well, first find where code is repeated. 80% of developers struggle with this24. Then, make parts of your code reusable and flexible.
Real examples show how DRY works. For instance, putting all discount rules in one place makes things simpler2625.
Using DRY has many benefits. It makes your code easier to keep up, read, and fix bugs26. It also makes updates less likely to cause problems.
By finding the right balance, you can use DRY to its fullest in your coding work.
Start using DRY to make your code better and more efficient. It will help you create code that lasts. Every line of code is a chance to show you care about coding well.
Leave a Comment