Is MATLAB really a programming language, or is it something different? Many have wondered this, especially with over 2,000,000 users in science1. MATLAB started in the mid-1980s by Cleve Moler and John Little1. It’s now key in fields like engineering and economics. But what is MATLAB, and how does it fit into programming?
Table of Contents
MATLAB is a special programming language and a place for numeric computing. It lets users work with matrices, plot data, and more. It’s easy to use because you can run commands right away1. This makes it great for those who want a simple, high-level language1.
We’ll explore MATLAB’s history, main features, and how it stacks up against other languages. We’ll look at its programming styles, data types, and how it handles functions and files. By the end, you’ll know if MATLAB is a programming language and how to use it in your projects.
What is MATLAB?
MATLAB is short for Matrix Laboratory. It’s a high-level programming language and a numerical computing environment. MathWorks developed it. It’s great for data analysis, algorithm development, and scientific computing.
Its syntax is easy to understand. It has lots of libraries. This makes MATLAB popular among engineers, scientists, and researchers.
History and Development of MATLAB
Cleve Moler created MATLAB in the late 1970s. It started as a simple matrix calculator. In 1984, it was first sold at the Automatic Control Conference2.
The first sale was in 19852. By the late 1980s, hundreds of universities bought it for students2. Version 3 came out in 19872.
Over time, MATLAB grew a lot. In 2000, MathWorks added a Fortran-based library for linear algebra in MATLAB 62. The Parallel Computing Toolbox was released in 20042.
In 2010, MATLAB started supporting graphics processing units (GPUs)2. Version 8 was released in 20122. By 2016, it had many technical and user interface improvements2.
Key Features of MATLAB
MATLAB has many features for scientific computing and data analysis. Some key features are:
- Intuitive syntax for matrix and array mathematics
- Thousands of built-in functions for calculations
- Extensive plotting and visualization capabilities
- Integration with other programming languages through MATLAB Engine APIs3
- Ability to call libraries from different programming languages3
MATLAB is easy to use and versatile. As of 2020, it has over four million users worldwide2. It’s used in colleges, universities, startups, and big companies like Apple and Google4.
By 2017, over 5000 colleges and universities used MATLAB2. About 8 million people have access to MATLAB through their school or job4.
MATLAB as a Programming Language
MATLAB is more than just a tool for math; it’s a full-fledged programming language. It’s used by millions of engineers and scientists for data analysis and model creation5. It combines a desktop environment with a language that directly expresses matrix and array mathematics5. It supports various programming paradigms, including functional, imperative, procedural, and object-oriented programming6.
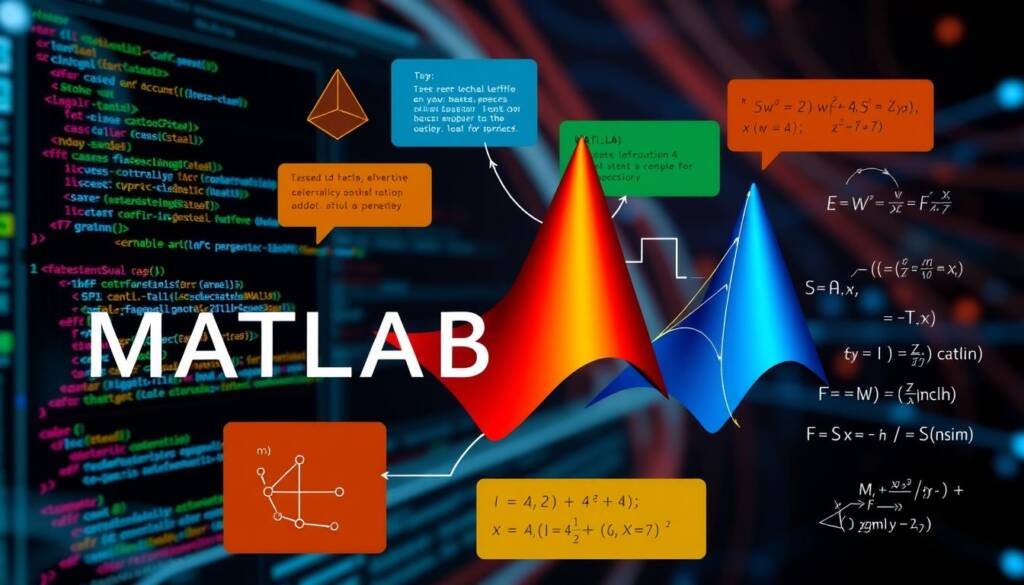
MATLAB Syntax and Basics
MATLAB’s syntax is easy to learn, making it great for both new and experienced programmers. It has a function library with basic math functions like sin, cos, and tan. It also has complex expressions, such as matrix eigenvalues6.
Variables are defined using the assignment operator (=). MATLAB is a weakly typed language, meaning types are converted when needed.
MATLAB is great for quick numeric calculations and analysis7. It’s perfect for scientific computing, data analysis, and algorithm development6. It also provides better visualization of plots and data compared to other languages7.
Comparison with Other Programming Languages
MATLAB is unique compared to other programming languages. Unlike open-source languages like R, MATLAB is a proprietary program developed and maintained by MathWorks7. However, MATLAB has advantages like quicker execution of basic programming functions and a comprehensive set of toolboxes for various applications7.
In analytics, R is often preferred over MATLAB for its strengths in statistical analysis and machine learning libraries7. But MATLAB balances statistical analysis with other domains like control systems and deep learning57.
Language | Strengths | Weaknesses |
---|---|---|
MATLAB | Numeric computation, visualization, toolboxes | Proprietary, cost |
R | Statistical analysis, machine learning, open-source | Slower for basic programming functions |
Python | General-purpose, open-source, extensive libraries | Slower than MATLAB for numeric computation |
The choice between MATLAB and other programming languages depends on the project’s needs, the user’s expertise, and resources. MATLAB’s versatility, performance, and toolboxes make it a top choice for engineers and scientists6.
MATLAB Programming Paradigms
MATLAB is a versatile programming language. It supports many programming paradigms. This makes it great for different coding styles and solving problems.
The matlab programming environment lets users use various techniques. They can use functional, imperative, procedural, object-oriented, and array programming. This helps in creating efficient and effective matlab code8.
MATLAB is fast for developing algorithms compared to languages like C, C++, or Fortran. It doesn’t need users to declare variables or compile code. This makes coding faster8.
Functional programming in MATLAB makes code concise and easy to read. It treats computation as evaluating mathematical functions. This is great for complex math and data manipulation.
Imperative and procedural programming in MATLAB lets users write step-by-step instructions. It’s good for tasks needing detailed commands and control flow.
MATLAB also supports object-oriented programming (OOP). OOP organizes code into objects with data and behavior. It helps in code reuse, modularity, and creating scalable apps.
MATLAB’s array programming is very powerful. It allows operations on arrays or matrices without loops. This makes code concise and efficient.
MATLAB is widely used in industries like oil, automotive, biosciences, and defense. It’s great for scientific and engineering computing8. Its versatility and supported paradigms make it popular among professionals and researchers.
To get the most out of MATLAB, it’s important to practice. There are many learning resources. These include official MATLAB documentation, online courses, and books8.
In summary, MATLAB’s support for various paradigms and ease of use make it essential. It’s great for solving complex problems and developing efficient algorithms. This solidifies its role as a top choice for scientific and engineering applications.
Data Types in MATLAB
MATLAB has many data types for different kinds of data. These types are key to learning MATLAB. There are 16 main data types, like numbers, text, and more9. Each type has special properties and functions for specific tasks.
Numeric Data Types
Numeric types in MATLAB handle numbers and math. It has types like double-precision floating-point and integers. You can check a variable’s type with isinteger, isfloat, and isnumeric910.
Character and String Data Types
Character and string types store text. MATLAB has char for single characters and string for text sequences. You can use ischar to see if a variable is text910.
Structures and Cell Arrays
Structures and cell arrays help organize different data types. Structures group related data, and cell arrays hold various data types9.
Structures are like folders for data, and you use dot notation to access them. MATLAB’s isstruct function checks if something is a structure910.
Cell arrays are flexible containers for different data sizes and types. You can access elements with curly braces {}.
Data Type | Description |
---|---|
Numeric | Represents numbers and performs mathematical operations |
Character and String | Stores and manipulates text data |
Structures | Groups related data using fields |
Cell Arrays | Stores data of different sizes and types within a single array |
MATLAB has functions for changing data types, like mat2str and str2num10. These help work with different data types smoothly.
Knowing MATLAB’s data types is key for good programming and data handling.
Using the right data types and functions makes your code better. This makes your MATLAB programs more solid and easy to keep up.
Variables and Operations in MATLAB
In MATLAB, you use the assignment operator (=) to define variables. They are case-sensitive. You can assign values in the Command Window, which is used all the time11. For example, you can make a new variable x = 55 in MATLAB. The output will show the variable’s value11.
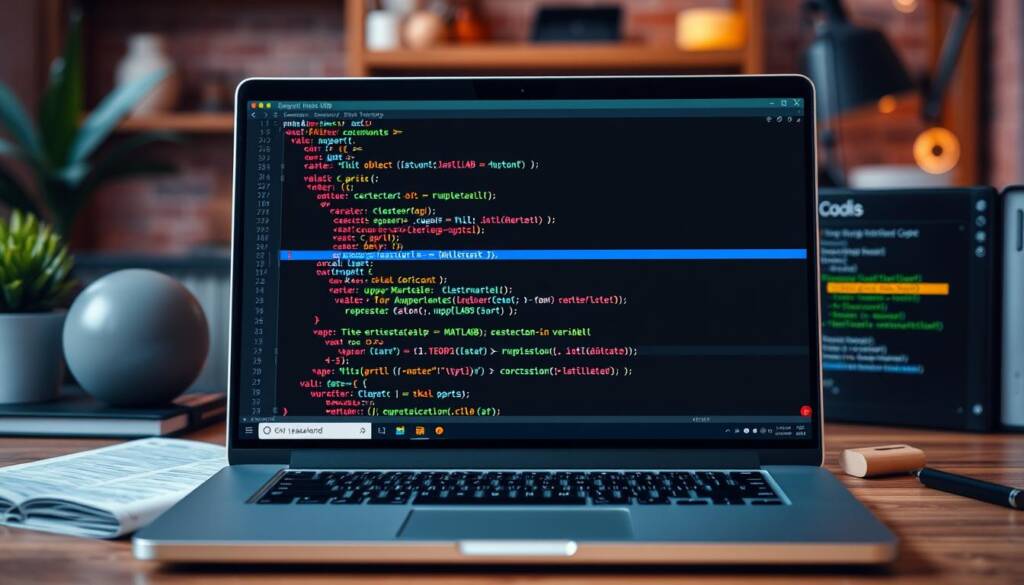
MATLAB has many data types. Numbers are saved as double, letters as ‘char’, and words as strings. Logical values are 0 or 111. You can make variables for weight, size3, patient_name, and alive_on_day_3 with their values in MATLAB11.
MATLAB has a specific order for arithmetic operations, as shown in the table below12:
Operation | Precedence |
---|---|
Parentheses | Highest |
Exponentiation | Second Highest |
Multiplication and Division | Third Highest |
Addition and Subtraction | Lowest |
You can do simple things like add and multiply in MATLAB. For example, x + y = 65 is a simple sum. Z = x * y = 550 shows multiplication11.
Arrays and matrices are key in MATLAB. You make an array with square brackets and semi-colons. For example, Array A is [1, 2, 3], a 1×3 array. Matrix B is [1, 2; 3, 4; 5, 6], a 3×2 matrix11. You can also make arrays with different data types11.
Knowing arrays is key for good programming in MATLAB. Most functions work with arrays and do things to each element11.
MATLAB is designed for solving problems and easy programming. It’s great at linear algebra, often better than FORTRAN or C13. You can do many things with arrays in MATLAB, like checking values, summing, and multiplying11.
Functions and File Organization in MATLAB
MATLAB is great for making and using functions. It also helps you organize your files well. Functions in MATLAB are more useful than scripts. They let you do specific tasks and use code again easily14.
Creating and Using Functions
To make a function in MATLAB, follow the right syntax and rules. The file name should match the first function’s name. Names should start with a letter, then letters, numbers, or underscores14.
Here’s an example of turning a script into a function. It calculates a triangle’s area:
b = 5;
h = 3;
area = 0.5 * b * h;
disp(area);
This script finds a triangle’s area with a base of 5 and height of 3. It shows an area of 7.514. By making it a function named triarea
, you can easily find areas of different triangles14. The function’s workspace is separate, making your code cleaner and more organized14.
MATLAB File Types and Organization
MATLAB has many file types for different uses. The main types are:
.m
files for MATLAB code.mat
files for binary data.fig
files for figures.mlx
files for live scripts
It’s important to organize your MATLAB files well. Keep your code in separate libraries in their own folders, as most developers suggest15. Keep your code and control files in the same folder for others to use later15. Don’t hard-code directory or file names, as most developers advise15.
For better project management, keep your MATLAB code near other languages’ code15. Use code that finds directories and adds paths as needed15. This makes your projects more organized and easy to work on.
Object-Oriented Programming in MATLAB
MATLAB is a top programming language and environment. It supports object-oriented programming (OOP). This lets users make strong and easy-to-use code.
With OOP, you can make your code better. It becomes easier to use and keep up with. You can make objects that act like real things16.
It also makes your code simpler. You can organize it into parts that make sense16.
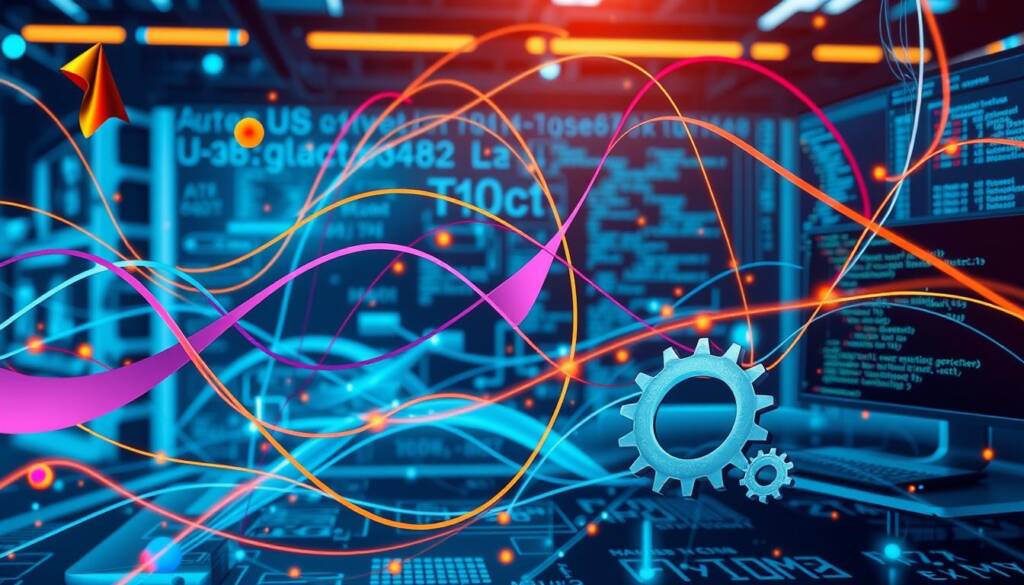
Defining Classes and Objects
In MATLAB, classes are like blueprints for objects. A class has properties, methods, and events16. Properties are the data, methods are the actions, and events are how objects talk to each other.
Objects are specific instances of classes. They have their own data and actions17. MATLAB takes care of the objects without you needing to worry about memory16.
Inheritance and Polymorphism in MATLAB
MATLAB lets you create class relationships. This means you can make a base class and then special classes from it17. The special classes get the base class’s stuff but can also do their own things.
Polymorphism is key in MATLAB OOP. It lets different classes act like they’re the same17. This makes your code more flexible and reusable.
Objects in MATLAB have cool features. They can check data, calculate values, and control who can see them17. This makes your objects strong and safe.
MATLAB classes have different levels of access. This helps keep your code organized and safe17. You can also make special ways for objects to interact with each other17.
Using OOP in MATLAB makes your code better. It’s easier to use and keep up with. Objects hide the complicated stuff and make your code work well together17.
Let’s say you want to analyze sensor data. You can use MATLAB OOP to make it easier. Here’s how:
- Make a class for the sensor array. It should have things like how many sensors there are and how fast light goes18.
- Add methods to the class for actions like finding where signals come from18.
- Use special methods to make the analysis better18.
- Make sure you can get and set properties in the class18.
- Make a special plot method for your object18.
By following these steps, you can make a great application for sensor data. OOP makes your code flexible and easy to change for different needs.
In summary, MATLAB OOP is great for making good code. You can define classes, create objects, and use inheritance and polymorphism. This makes your code better for many areas like science and control systems.
Toolboxes and Libraries in MATLAB
MATLAB has many toolboxes and libraries. They make it great for things like signal and image processing, control systems, and more19. These tools help users solve tough problems easily.
- Signal Processing Toolbox
- Image Processing Toolbox
- Control System Toolbox
- Statistics and Machine Learning Toolbox
- Optimization Toolbox
- Symbolic Math Toolbox
These toolboxes use MATLAB’s strong points to help users write clear code19. They also have great documentation. This makes it easy for users to learn and use the tools19.
Toolbox | Key Features |
---|---|
Signal Processing Toolbox | Filter design, spectral analysis, signal generation |
Image Processing Toolbox | Image enhancement, segmentation, feature extraction |
Control System Toolbox | Linear and nonlinear control system design and analysis |
Statistics and Machine Learning Toolbox | Data analysis, regression, classification, clustering |
MATLAB’s toolboxes are easy to use because they are consistent19. This, along with its ability to handle big data, makes it a top choice for many19.
MATLAB’s toolboxes and libraries have been key in pushing research and development forward. They give experts the tools they need to solve complex problems and speed up innovation.
Using MATLAB’s toolboxes and libraries can really boost your work. It makes MATLAB a must-have for many fields in science and engineering.
Applications of MATLAB Programming
MATLAB is a versatile programming language used in many fields. It helps professionals and researchers solve complex problems. Its strong computing power and wide range of tools make it very useful20.
Scientific Computing and Numerical Analysis
In scientific computing and numerical analysis, MATLAB is a top choice. It’s great for solving tough math problems. Its matrix-based operations and libraries work fast, especially on computers with many cores21.
MATLAB’s profiler helps improve program performance. It shows how long functions take to run and how often they’re called. This helps make code better21.
Signal and Image Processing
MATLAB is also excellent for signal and image processing. It has built-in algorithms and toolboxes that make work easier21. It can handle signal filtering, transformation, and image analysis. This is very helpful for researchers and engineers20.
Control Systems and Robotics
In control systems and robotics, MATLAB is a key tool. It’s great for modeling, simulating, and designing complex systems. It works well with Simulink for creating and testing control algorithms20.
MATLAB also supports parallel computing. This means it can run tasks faster by using many computers at once. This is very helpful for big tasks21.
Even though MATLAB isn’t always mentioned in job ads, knowing it can still get you hired22. Employers value MATLAB skills, especially in research and development. They care more about problem-solving skills than the programming language22.
MATLAB’s wide-ranging applications and industry-specific toolboxes make it an indispensable asset for professionals seeking to excel in their respective fields.
Learning Resources for MATLAB Programming
Starting to learn MATLAB programming? You’ll find lots of resources to help you. There are official guides, tutorials, online courses, and books for all levels and learning styles.
Official MATLAB Documentation and Tutorials
Begin with MathWorks’ official resources. The MATLAB Onramp tutorial is great for beginners. It introduces MATLAB basics and syntax23. The MathWorks website also has many tutorials for deeper learning23.
Online Courses and Tutorials
There are many online courses and tutorials to learn MATLAB. MathWorks has a 10-minute video on getting started with MATLAB24. They also have courses on data processing and deep learning24. The official MATLAB YouTube Channel has playlists on advanced topics and machine learning24.
Try the MATLAB Onramp course by MathWorks for a structured learning experience24. MIT Open Courseware offers an undergraduate course on MATLAB, covering the basics24.
Books and Reference Materials
Books and reference materials are also great for learning MATLAB. “MATLAB for Beginners” by Peter Kattan is perfect for new learners24. “MATLAB Programming for Engineers” by Stephen J. Chapman is great for advanced learners24.
Learning MATLAB well requires a strong math background. You’ll need knowledge in discrete math, linear algebra, probability, and statistics23. As you learn, apply what you know to real tasks. Don’t look for answers right away. This will help you understand better23.
Conclusion
MATLAB is a strong and flexible programming language. It’s used a lot in science, engineering, and economics. It has special features like matrix handling and lots of tools, making it key for many professionals and students25.
It’s different from other programming languages in some ways. But, it’s great for solving tough problems26.
MATLAB is popular because it’s easy to use and solves math problems well. It’s used a lot in schools, work, and research25. It helps people work faster in science and engineering.
Also, you can use MATLAB Online from any web browser. This means you don’t need to install software on your computer25.
If you want to learn MATLAB, there are many resources. MathWorks offers official guides, tutorials, and courses25. With these, you can learn MATLAB fast and solve real problems.
Starting with MATLAB programming opens up new ways to solve problems and analyze data. It can change how you work in your field.
Leave a Comment